|
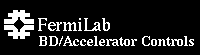

Sequential Delete
This example demonstrates how to delete records sequentially from an APPDS table. In the code the appds_delete_record routine is called twice, first to delete the first three records and then again to delete the next three records. Critical elements are shown in bold.
#include <stdio.h>
#include "clib.h"
#include "acnet_errors.h"
#include "appds.h"
main()
{
int records_to_delete = 3;
int records_deleted;
char error_text[512];
int return_code; /* ACNET return code */
/* delete the first 3 records */
return_code = appds_delete_record("MY_TABLE",(void *) NULL,records_to_delete,
&records_deleted,error_text,"APPDB","ADBS");
if (return_code == APPDS_SUCCESS)
{
printf("deleted %d records\n");
} else {
printf("ERROR:%s\n",error_text);
}
/* delete the next 3 records */
return_code = appds_delete_record("MY_TABLE",(void *) NULL,records_to_delete,
&records_deleted,error_text,"APPDB","ADBS");
if (return_code == APPDS_SUCCESS)
{
printf("deleted %d records\n");
} else {
printf("ERROR:%s\n",error_text);
}
}
|
Indexed delete
This example demonstrates how to delete records via indexes from an APPDS table. In the code the appds_delete_record routine is called twice, first to delete the records who's record Id's are [1,4,6] and then again to delete the records with Id's [10,22,45]. Critical elements are shown in bold.
#include <stdio.h>
#include "clib.h"
#include "acnet_errors.h"
#include "appds.h"
main()
{
int records_to_delete = 3;
int records_deleted;
char error_text[512];
int return_code; /* ACNET return code */
long id_array[3];
/* delete the first 3 records */
id_array[0]=1;
id_array[1]=4;
id_array[2]=6;
return_code = appds_delete_record("MY_TABLE",id_array,records_to_delete,
&records_deleted,error_text,"APPDB","ADBS");
if (return_code == APPDS_SUCCESS)
{
printf("Deleted %d records\n",records_deleted);
} else {
printf("ERROR:%s\n",error_text);
}
/* delete the next 3 records */
id_array[0]=10;
id_array[1]=22;
id_array[2]=45;
return_code = appds_delete_record("MY_TABLE",,id_array,records_to_delete,
&records_deleted,error_text,"APPDB","ADBS");
if (return_code == APPDS_SUCCESS)
{
printf("Deleted %d records\n",records_deleted);
} else {
printf("ERROR:%s\n",error_text);
}
}
|
Keyed delete
This example demonstrates how to delete records via a key from an APPDS table. In the code the appds_delete_record routine is called passing "FERMI" for the key value. Critical elements are shown in bold.
#include <stdio.h>
#include "clib.h"
#include "acnet_errors.h"
#include "appds.h"
main()
{
int not_used_variable;
int records_deleted;
char error_text[512];
int return_code; /* ACNET return code */
char key_value = "FERMI";
return_code = appds_delete_recod("MY_TABLE",key_value,not_used_variable,
&records_deleted,error_text,"APPDB","ADBS");
if (return_code == APPDS_SUCCESS)
{
printf("Deleted %d records\n");
} else {
printf("ERROR:%s\n",error_text);
}
}
|
webmaster@adwww.fnal.gov
Last modified: Fri Feb 12 16:51:40 CST 1999
|