|
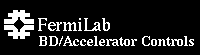

Sequential write
This example demonstrates how to write records sequentially to an APPDS table. In the example the appds_write_record routine is called twice, first to update the first record and then again to add 3 records. Critical elements are shown in bold.
#include <stdio.h>
#include "clib.h"
#include "acnet_errors.h"
#include "appds.h"
struct NAME_STRUCT {
char *last_name;
char *first_name;
};
main()
{
struct NAME_STRUCT record_array[3];
struct NAME_STRUCT one_record;
int records_to_write;
char error_text[512];
int return_code; /* ACNET return code */
/* Update first record */
sprintf(one_record.first_name,"ENRICO");
sprintf(one_record.last_name,"FERMI");
records_to_write=1;
return_code = appds_write_record("MY_TABLE",APPDS_UPDATE,records_to_write,
&one_record,(void *) NULL,error_text,"APPDB","ADBS");
if (return_code == APPDS_SUCCESS)
{
printf("Added %d records to the table.\n",records_to_write);
} else {
printf("ERROR:%s\n",error_text);
}
/* Add 3 records */
sprintf(record_array[0].first_name,"ENRICO");
sprintf(record_array[0].last_name,"FERMI");
sprintf(record_array[1].first_name,"ENRICO");
sprintf(record_array[1].last_name,"FERMI");
sprintf(record_array[2].first_name,"ENRICO");
sprintf(record_array[2].last_name,"FERMI");
records_to_write=3;
return_code = appds_write_record("MY_TABLE",APPDS_ADD,records_to_write,
record_array,(void *) NULL,error_text,"APPDB","ADBS");
if (return_code == APPDS_SUCCESS)
{
printf("Updated %d records.\n",records_to_write)
} else {
printf("ERROR:%s\n",error_text);
}
}
|
Indexed write
This example demonstrates how to write records to an APPDS table via indexes. In the code the appds_write_record routine is called twice, first to add 3 records and then again to update the records with Id's [10,22,45]. Critical elements are shown in bold.
#include <stdio.h>
#include "clib.h"
#include "acnet_errors.h"
#include "appds.h"
struct NAME_STRUCT {
char *last_name;
char *first_name;
};
main()
{
struct NAME_STRUCT record_array[3];
int records_to_write = 3;
char error_text[512];
int return_code; /* ACNET return code */
long id_array[3];
/* Load the record array */
sprintf(record_array[0].first_name,"ENRICO");
sprintf(record_array[0].last_name,"FERMI");
sprintf(record_array[1].first_name,"ENRICO");
sprintf(record_array[1].last_name,"FERMI");
sprintf(record_array[2].first_name,"ENRICO");
sprintf(record_array[2].last_name,"FERMI");
records_to_write=3;
/* Add 3 records */
return_code = appds_write_record("MY_TABLE",APPDS_ADD,records_to_write,
record_array,(void *) NULL,error_text,"APPDB","ADBS");
if (return_code == APPDS_SUCCESS)
{
printf("Added %d records\n",records_to_write);
} else {
printf("ERROR:%s\n",error_text);
}
/* Update 3 records */
id_array[0]=10;
id_array[1]=22;
id_array[2]=45;
return_code = appds_write_record("MY_TABLE",APPDS_UPDATE,records_to_write,
record_array,id_array,error_text,"APPDB","ADBS");
if (return_code == APPDS_SUCCESS)
{
printf("Updated %d records\n",records_to_write);
} else {
printf("ERROR:%s\n",error_text);
}
}
|
Keyed write
This example demonstrates how to write records on an APPDS table via a key value. In the code the appds_write_record routine is called twice, once to add 3 records and then again to update records with a key value of "SMITH". Critical elements are shown in bold.
#include <stdio.h>
#include "clib.h"
#include "acnet_errors.h"
#include "appds.h"
struct NAME_STRUCT {
char *last_name;
char *first_name;
};
main()
{
struct NAME_STRUCT record_array[3];
struct NAME_STRUCT update_record;
int records_to_write;
int records_returned;
char error_text[512];
int return_code; /* ACNET return code */
char key_value = "SMITH";
/* Load the record array */
sprintf(record_array[0].first_name,"ENRICO");
sprintf(record_array[0].last_name,"FERMI");
sprintf(record_array[1].first_name,"ENRICO");
sprintf(record_array[1].last_name,"FERMI");
sprintf(record_array[2].first_name,"ENRICO");
sprintf(record_array[2].last_name,"FERMI");
records_to_write=3;
/* Add 3 records */
return_code = appds_write_record("MY_TABLE",APPDS_ADD,records_to_write,
record_array,(void *) NULL,error_text,"APPDB","ADBS");
if (return_code == APPDS_SUCCESS)
{
printf("Added %d records\n",records_to_write);
} else {
printf("ERROR:%s\n",error_text);
}
/* Load update record */
sprintf(update_record.first_name,"ENRICO");
sprintf(update_record.last_name,"FERMI");
/* Update records */
return_code = appds_write_record("MY_TABLE",APPDS_UPDATE,records_to_write,
record_array,key_value,error_text,"APPDB","ADBS");
if (return_code == APPDS_SUCCESS)
{
printf("Updated %d records\n",records_to_write);
} else {
printf("ERROR:%s\n",error_text);
}
}
|
webmaster@adwww.fnal.gov
Last modified: Fri Feb 12 13:09:58 CST 1999
|